1. Introduction :
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient.
For more refer to my article :
1. All About Node.Js You Wanted To Know ?
2. Node.js Installation :
You need to follow these simple steps
Installation steps :
- Download the installer from the official Node.js site link : https://nodejs.org/en/download/ based on your machine OS.
- Run the installer. Follow the installer steps, agree the license agreement and follow the instructions by clicking next button.
- Restart your system/machine to finish the installation process .
If you need add more Node.js version you should use nvm: Node Version Manager . Choose your nvm based on your machine os.
Installing Node.js Versions
nvm install 5.5.1(use latest LTS version)
To verify that you have Node.js up and running, run this:
node --version
If everything is ok, it will return the version number of the currently active Node.js binary.
Using Node.js Versions
If you are working on a project supporting Node.js v4, you can start using it with the following command:
nvm use 4
Then you can switch to Node.js v5 with the very same command:
nvm use 5
In Node.js the stable versions with long-term support (LTS) are the ones starting with even numbers (4, 6, 8 …) and the experimental version are the odd numbers (5, 7 …). It is recommend you to use the LTS version in production and try out new things with the experimental one.
Node Package Manager :
Node Package Manager (NPM) provides two main functionalities −
- Online repositories for node.js packages/modules which are searchable on search.nodejs.org
- Command line utility to install Node.js packages, do version management and dependency management of Node.js packages.
NPM helps javascript developers load dependencies effectively. To load dependencies we just have to run a command in command prompt:
> npm install
This command is finding a json file named as package.json
in root directory to install all dependencies defined in the file.
Package.json
Package.json looks like:
{
"name": "ApplicationName",
"version": "0.0.1",
"description": "Application Description",
"main": "server.js",
"scripts": {
"start": "node server.js"
},
"repository": {
"type": "git",
"url": "https://github.com/npm/npm.git"
},
"dependencies": {
"express": "~3.0.1",
"sequelize":"latest",
"q":"latest",
"tedious":"latest",
"angular":"latest",
"angular-ui-router": "~0.2.11",
"path":"latest",
"dat-gui":"latest"
}
}
The most important things in your package.json are name and version. Those are actually required, and your package won’t install without them. The name and version together form an identifier that is assumed to be completely unique. Changes to the package should come along with changes to the version.
NPM provide many useful Scripts like npm install
, npm start
, npm stop
etc.
Some default script values are based on package contents.
"start": "node server.js"
If there is a server.js file in the root of your package, then npm will default the start command to node server.js.
Dependencies
{
"dependencies": {
"express": "~3.0.1",
"sequelize":"latest",
"q":"latest",
"tedious":"latest",
"angular":"latest",
"angular-ui-router": "~0.2.11",
"path":"latest",
"dat-gui":"latest"
}
}
Dependencies are specified in a simple object that maps a package name to a version range. Version Name must be Version exactly.
Writing Hello world Node.js Program :
A Node.js application involves following 3 steps :
- Import required modules − We use the require directive to load Node.js modules.
- Create server − A server which will listen to client’s requests similar to Apache HTTP Server.
- Read request and return response − The server created in an earlier step will read the HTTP request made by the client which can be a browser or a console and return the response.
Let’s go through this steps by an example :
Create a server.js: javascript file with following code
/*server.js*/const http = require('http');const hostname = '127.0.0.1';
const port = 3000;const server = http.createServer(function(req, res) {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
});server.listen(port, hostname, function() {
console.log('Server running at http://'+ hostname + ':' + port + '/');
});
As we need http
to create an http server we use require('http')
and pass it to a variable named http
- This is how we import required modules :
var http = require('http');
We also need to defined hostname and port number, here we use localHost
i.e 127.0.0.1
and port
number 3000(it can vary based on your preferences)
and assign this to the variables hostname
and port
, respectively.
var hostname = '127.0.0.1';
var port = 3000;
2. Creating Http Server :
Next we create the http server using the createServer
method.
var server = http.createServer(function(req, res){
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
});
This created the server as well as a response having statusCode: 200
, Content-Type
header of plain text and and ends with the string Hello World
. This is the response that the server can send to browser.
the function has two parameters req
and res
which is the request
from and response
to the server, respectively.
3. Read request and return response
As we created the server above , now it’s time to assign it a hostname and port number.
server.listen(port, hostname, function() {
console.log('Server running at http://'+ hostname + ':' + port + '/');
});
Here, the server listens to localhost on port 3000 and prints “Server running at http://127.0.0.1:3000/ “ in command prompt.
Now Run server.js file using command shown below
> node server
Type url http://127.0.0.1:3000/ in the browser, to display Hello World string as shown on the screen below :
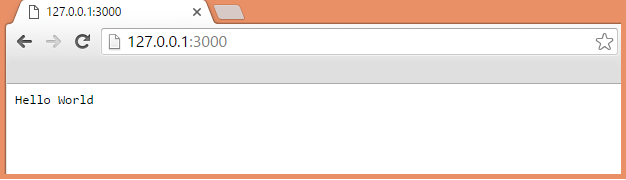
As always after some code basics let me give some facts of Node.js to help you understand why Node.js becoming so popular among corporates and developer community and why you should think about getting into it.
Learn Complete Node.js Tutorial in Myanmar Language Click these link -> Complete Tutorial Node.js